반응형
Truthy & Falsy
- 자바스크립트는 자료형 상태에따라 참 거짓(TRUE,FALSE)로 평가하는 자료형들이 있다.
const getName = (person) => {
if (person === undefined || person === null) {
return "객체가 아닙니다.";
}
return person.name;
};
let person = null;
const name = getName(person);
console.log(name);
이와같이 작성할 수 있는것을 아래와같이 간소화 가능하다.
const getName = (person) => {
if (!person) {
return "객체가 아닙니다.";
}
return person.name;
};
let person = null;
const name = getName(person);
console.log(name);
삼항연산자(조건문 한줄로 끝내기)
let a = -3;
a >= 0 ? console.log("양수") : console.log("음수");
let b = [1, 23];
b.length === 0 ? console.log("빈배열") : console.log("비지않은 배열");
let c = [];
const arrayStatus = c.length === 0 ? "빈배열" : "안빈배열";
console.log(arrayStatus);
let d = [];
const result = d ? true : false;
console.log(result);
음수
비지않은 배열
빈배열
true
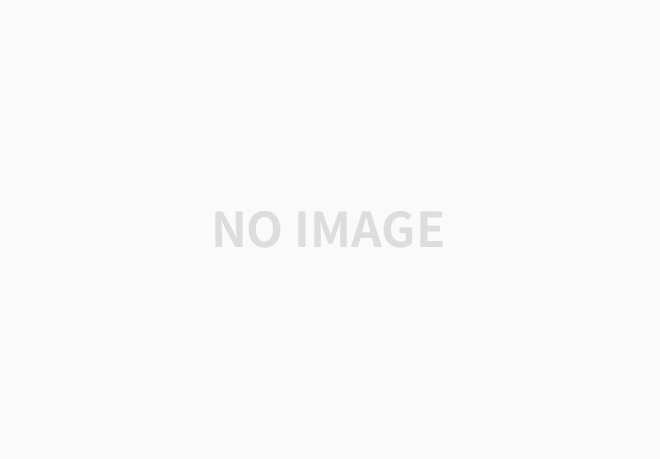
그리고 삼항연산자도 중첩이 가능하지만, 그런경우는 if문으로 사용해 주는게 좋다.
단락 회로 평가
왼쪽에서 오른쪽으로 진행하는 논리연산, true&false를 이용한다. (&&와 ||를 사용)
||는 앞이 참이면 바로 앞에 참을 출력한다.
const getName = (person) => {
const name = person && person.name; //null
return name || "객체가 아닙니다.";
};
let person; // null or undefiend다 넣어보기
const name = getName(person);
console.log(name); //
// let person = { name: "김지원" };
// const name = getName(person);
// console.log(name);
조건문 업그레이드(배열, 객체사용)
- 배열사용 (includes)
function isKoreanFood(food) {
if (["불고기", "떡볶이", "비빔밥"].includes(food)) {
return true;
}
return false;
}
const food1 = isKoreanFood("불고기");
const food2 = isKoreanFood("파스타");
console.log(food1, food2);
- 객체사용
const meal = {
한식: "불고기",
중식: "멘보샤",
일식: "초밥",
양식: "스테이크",
인도식: "카레"
};
const getMeal = (mealType) => {
return meal[mealType] || "굶기";
};
console.log(getMeal("한식"));
console.log(getMeal());
비 구조화 할당(구조분해할당) 배열과 객체에 사용한다.
// let [one, two, three] = ["one", "two", "three"];
// console.log(one, two, three);
// 순서대로 배열의 요소를 변수에 할당하는 방법
let [one, two, three, four = "four"] = ["one", "two", "three"];
console.log(one, two, three, four);
//기본값을 안 지정하면 undefined
- 스왑하기 (swap)
let a = 10;
let b = 20;
let tmp = 0;
tmp = a;
a = b;
b = tmp;
console.log(a, b);
이와같은 문법을 비구조화 할당으로 더 쉽게 가능하다.
let a = 10;
let b = 20;
[a, b] = [b, a];
console.log(a, b);
그리고 여러본 해본결과 비구조화 할당때 ; (세미콜론)을 제대로 쓰지않으면 오류가난다. 이거는 세미콜론 관련하여
다시 공부한걸 작성해보자, 동작원리가 문제인데
let a = 10
let b = 20
;[a, b] = [b, a]
console.log(a, b)
//세미콜론
이와같이 사용하면 문제가 없다, 하지만 에디터에 따라 위와같이 다시 바꿔준다.
멀쩡한데도 작동이 안되면 세미콜론을 의심해보자!
- 객체
let object = { one: "one", two: "two", three: "three" };
let one = object["one"];
let two = object.two;
let three = object.three;
console.log(one, two, three);
이와같이 할당하는걸 더 쉽게 사용 할 수 있다.
1) 객체 전체를 할당
let object = { one: "one", two: "two", three: "three" };
let { one, two, three } = object;
console.log(one, two, three);
//
2) 객체 키값을 기준으로 할당 (순서도 상관이 없다)
let object = { one: "one", two: "two", three: "three", name: "김지원" };
let { name, one, two, three } = object;
console.log(one, two, three, name);
3) 변수바꾸기, 기본값 할당까지 가능하다.
let object = { one: "one", two: "two", three: "three", name: "김지원" };
let { name: myName, one: oneOne, two, three, abc = "four" } = object;
console.log(oneOne, two, three, myName, abc);
spread연산자 (배열과 객체를 한줄로 펼치는 방법)
const cookie = {
base: "cookie",
madeIn: "korea"
};
const chocoCookie = {
...cookie,
toping: "chocochip"
};
const blueberyyCookie = {
...cookie,
toping: "blueberry"
};
console.log(chocoCookie, blueberyyCookie);
이와같이 공통항목을 가져올 수 있다. (중복요소)
- 배열에 사용할 때 ...연산자 [...다른배열]
// 배열에 사용할 떄
const noTopingCookies = ["촉촉한쿠키", "안촉촉한쿠키"];
const topingCookies = ["바나나쿠키", "블루베리쿠키", "딸기쿠키", "초코칩쿠키"];
const allCookies = [...noTopingCookies, ...topingCookies];
console.log(allCookies);
// concat과는 다르게 중간에 값도 넣을 수 있다.
const allCookies2 = [...noTopingCookies, "함정쿠키", ...topingCookies];
console.log(allCookies2);
복사를 해오는것이기도 하다. React같은곳에서 배열 복사에도 사용하고 그외 배열에 복사할 항목이 있을 때
...을 사용한다.
반응형
'Javascript' 카테고리의 다른 글
호이스팅(hoisting) (0) | 2023.03.27 |
---|---|
동기 & 비동기 - 1 (실행방식,콜백지옥) (0) | 2022.07.05 |
유용한 자바스크립트 문법 기본 (0) | 2022.06.23 |
Virtual DOM(가상돔) (0) | 2022.04.07 |
브라우저 렌더링 원리 (0) | 2022.04.07 |